The Complete Data Structures and Algorithms Course in Python
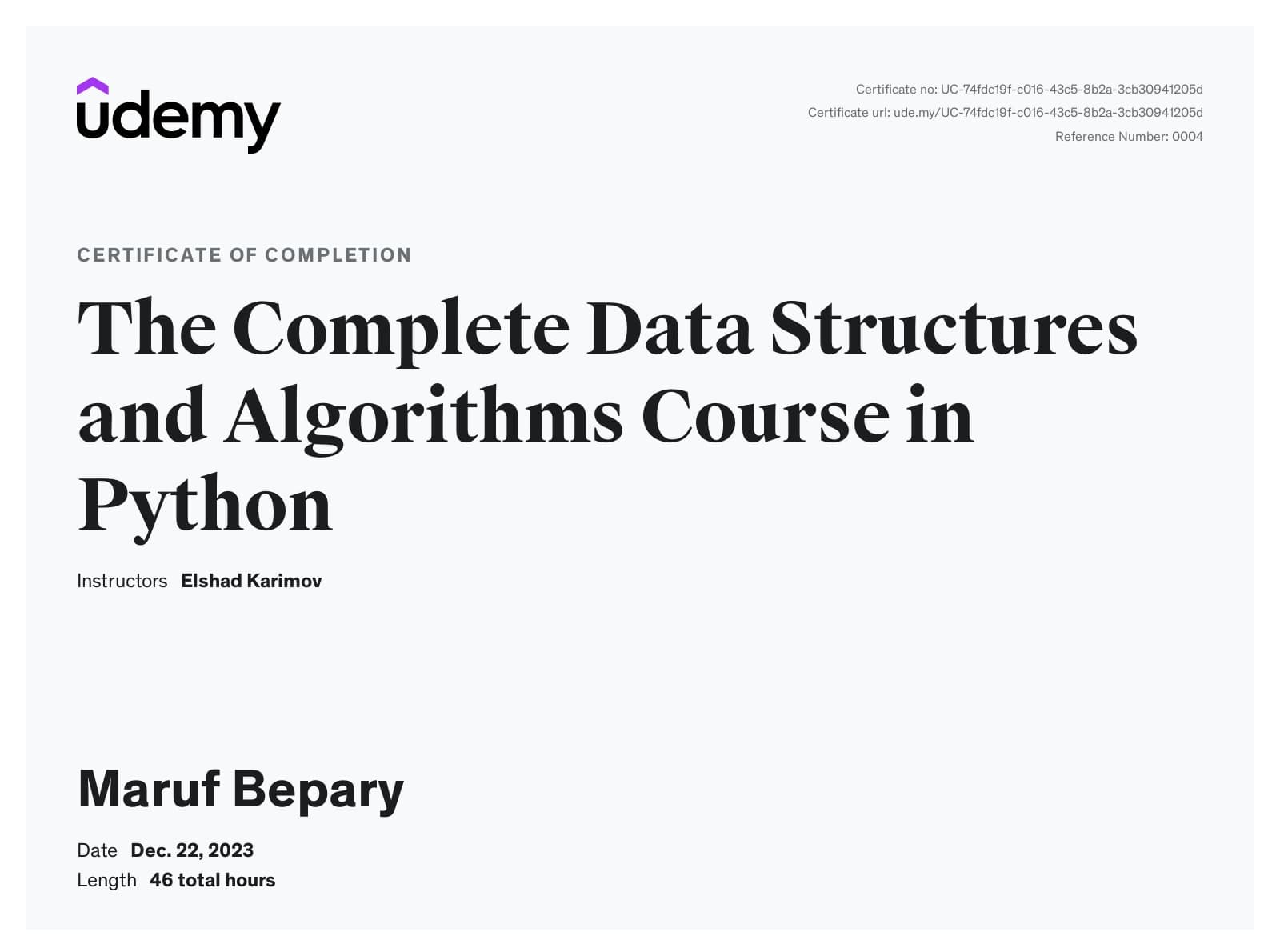
UC-74fdc19f-c016-43c5-8b2a-3cb30941205d
Description
This comprehensive Python Bootcamp offers a deep dive into Data Structures and Algorithms, covering essential topics and interview preparation for top tech companies. The course progresses from basic to advanced concepts, equipping learners with the skills to excel in professional programming and technical interviews. Tailored for a diverse audience, from self-taught programmers to seasoned developers, it provides lifetime access to extensive learning materials and a supportive community, all designed to boost career prospects in the tech industry.
Learning Objectives
- Understanding the role and purpose of data structures
- Grasping the fundamentals of recursion
- Solving common recursive problems
- Mastering recursion through intensive exercises
- Interpreting Big O notation for algorithm efficiency
- Preparing for complex Big O notation questions
- Understanding array structures and types
- Exploring list creation and manipulation in Python
- Tackling challenging array and list-based questions
- Enhancing skills with advanced array and list exercises
- Understanding dictionaries and their application
- Grasping the basics and utilities of tuples
- Differentiating types of linked lists
- Understanding and implementing circular singly linked lists
- Exploring the creation and manipulation of doubly linked lists
- Mastering circular doubly linked list operations
- Solving complex linked list problems in interviews
- Understanding stack data structure and its applications
- Grasping the fundamental concepts of queues
- Addressing common interview questions on stacks and queues
- Understanding tree structures and binary trees
- Exploring the creation and manipulation of binary search trees
- Understanding AVL trees and balancing factors
- Grasping the concept of binary heaps
- Understanding the structure and purpose of tries
- Exploring hashing concepts and hash functions
- Understanding various sorting techniques
- Grasping the fundamentals of search algorithms
- Understanding the concepts and types of graphs
- Comprehending the strategy behind greedy algorithms
- Understanding the divide and conquer paradigm
- Grasping the concept of dynamic programming
- Tackling intensive dynamic programming challenges